本篇介紹 Python 寫入二進制檔的方法,
以下 Python 寫入 binary 檔的方法將分為這幾部份,
- Python 寫入二進制檔的基本用法
- Python 使用
struct.pack()
寫入 str 字串到二進制檔 - Python 使用
struct.pack()
寫入 int 整數到二進制檔 - Python 使用
struct.pack()
寫入多種資料到二進制檔
Python 寫入二進制檔的基本用法
這邊介紹 Python 寫入二進制檔的基本用法,Python 寫二進制檔時 open()
開檔要使用 'wb'
,這邊示範寫入一個 hello 字串到二進制檔裡,write()
只接受 bytes 類別不接收 str 類別,所以需要將字串先轉成 bytes,1
2
3
4
5#!/usr/bin/env python3
# -*- coding: utf-8 -*-
with open('xxx.bin', 'wb') as f:
f.write(b'hello')
Ubuntu 下看二進制檔的 hex 的話可以使用 dhex 工具,使用 sudo apt install dhex
安裝即可使用,dhex 使用畫面如下圖所示,
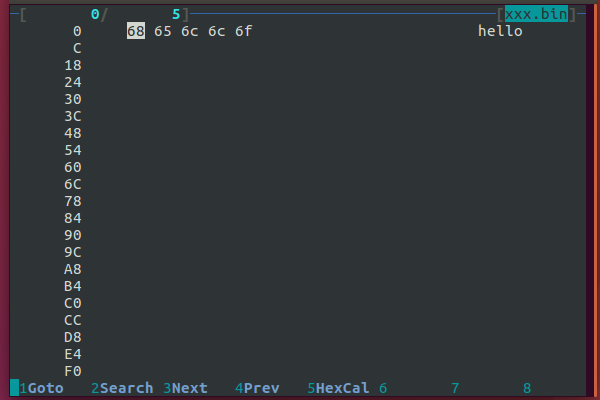
Python 使用 struct.pack()
寫入 str 字串到二進制檔
這邊介紹 Python 使用 struct.pack()
寫入 str 到二進制檔,Python 只提供 read 與 write 函式寫入,並沒有提供對二進制讀取與寫入的函式,但是可以透過 struct 模組來達成這件事,這邊使用 struct.pack()
將資料打包成 bytes 物件的形式然後透過 write()
寫入二進制檔,例如將 hello world
寫到二進制檔裡,1
2
3
4
5
6
7
8
9
10#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import struct
s = b'hello world'
bytes_str = struct.pack('11s', s)
print(type(bytes_str))
print(bytes_str)
with open('xxx.bin', 'wb') as f:
f.write(bytes_str)
如果要讀取的話可以看這篇。
Python 使用 struct.pack()
寫入 int 整數到二進制檔
這邊介紹 Python 使用 struct.pack()
寫入 int 到二進制檔,例如將 123
寫到二進制檔裡,1
2
3
4
5
6
7
8
9
10#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import struct
num = 123
bytes_str = struct.pack('i', num)
print(type(bytes_str))
print(bytes_str)
with open('xxx.bin', 'wb') as f:
f.write(bytes_str)
結果輸出如下,1
2<class 'bytes'>
b'{\x00\x00\x00'
如果要讀取的話可以看這篇。
Python 使用 struct.pack()
寫入多種資料到二進制檔
這邊介紹 Python 使用 struct.pack()
寫入多種資料到二進制檔,如果我們要 Python 使用 struct.pack()
寫入多種資料型態的話,可以這樣寫,假設我要寫入一個整數 123、一個浮點數 45.67,一個短整數 89,1
struct.pack('ifh', 123, 45.67, 89)
那麼將這些多種類型資料利用 struct.pack()
寫入二進制,就會是像這樣寫,1
2
3
4
5
6#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import struct
with open('xxx.bin', 'wb') as f:
f.write(struct.pack('ifh', 123, 45.67, 89))
剛剛是數字混合類型的範例,這次我們加入字串會是怎樣寫呢?
假設我要寫入一個字串 hello
、一個整數 12、一個浮點數 34.56、一個字串 python
,1
struct.pack('5sif6s', 'hello', 12, 34.56, 'python')
那麼將這些多種類型資料利用 struct.pack()
寫入二進制,就會像下範例這樣寫,1
2
3
4
5
6
7#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import struct
bytes_str = struct.pack('5sif6s', b'hello', 12, 34.56, b'python')
with open('xxx.bin', 'wb') as f:
f.write(bytes_str)
如果要讀取的話可以看這篇。
以上就是 Python 寫入二進制檔介紹,
如果你覺得我的文章寫得不錯、對你有幫助的話記得 Facebook 按讚支持一下!
其他參考
Python how to write to a binary file? - Stack Overflow
https://stackoverflow.com/questions/18367007/python-how-to-write-to-a-binary-file
其它相關文章推薦
Python 新手入門教學懶人包